[Javascript] 元のエラーを渡せる Error Cause
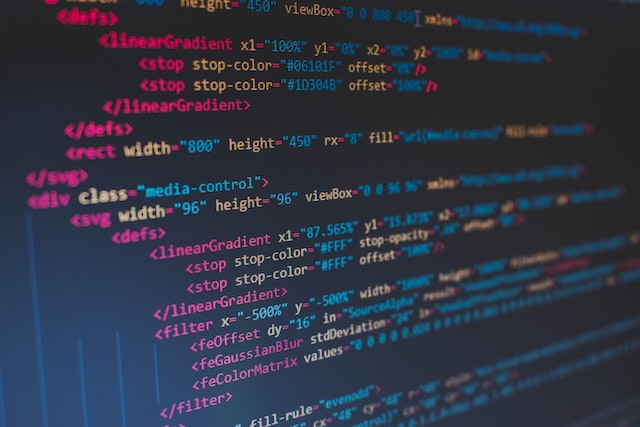
ES2022 で Error に cause がオプションとして追加され、再スローするときに元のエラーを渡すことができます。
以下、MDNのドキュメントより。
https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Error/cause
サンプル
fetch でエラーになるサンプルを作りました。
function UserException(message, options) {
this.message = message;
this.name = 'UserException';
this.cause = options.cause;
}
async function test() {
try {
const response1 = await fetch('http://example.co.jp/')
.catch(err => {
throw new UserException('fetch1回目でエラー', { cause: err });
});
const text1 = response1.text();
const response2 = await fetch('http://example.co.jp/', { method: 'POST', body: text1 })
.catch(err => {
throw new UserException('fetch2回目でエラー', { cause: err });
});
return response2.json().catch(err => {
throw new UserException('json()でエラー', { cause: err });
});
} catch (err) {
throw new Error('エラーです', { cause: err } );
}
}
test().catch(err => {
console.log(err);
console.log(err.cause);
})
console.log に出力された内容
fetch2回目でエラーになった場合の Devtools の表示
// Error: エラーです at test (sample:24:9)
// UserException {message: 'fetch2回目でエラー', name: 'UserException', cause: TypeError: Failed to fetch at test (sample:16:27)}
response2.json() で失敗した場合
// UserException {message: 'json()でエラー', name: 'UserException', cause: SyntaxError: Unexpected token 'T', "Text" is not valid JSON}
// SyntaxError: Unexpected token 'T', "Text" is not valid JSON
cause に渡すことで再スローされた先で簡単に元のエラーを受け取ることができました。
エラーの再スロー自体は一般的ですが、ES2022 以前には元のエラーを得る手段が標準で用意されていなかったのが不思議なくらいです。
エラー処理には悩まされることがよくあり、その場で処理することが難しい場合やまとめて処理しても問題ない場合などに使えそうです。
●●●
余談ですが、ブラウザによっては、cause とは別に stack が実装されているようですが、こちらは標準化はされなかったようです。stack という文字から Stack trace 的なものが格納されるのでしょうが、サーバーでの処理と違ってクライアント側なのでデバッグ後の消し忘れによる事故が多そうではあります。